In this post, we will make many menu-driven programs for different tasks, like a program to calculate the area of different shapes, perform the given tasks, and perform arithmetic operations between two matrices.
Menu-driven program in c: A program that obtains choice from a user by displaying the menu.
Here are three menu-driven programs using switch case, do-while loop, and while loop.
Table of Contents
Menu-driven program in c using Switch Case
In this program, we will create a menu-driven program that will print the area of different shapes according to the user's choice.
#include<stdio.h> int main() { int choice, radius, length, breadth, side; float area; printf("\n Press 1 to calculate area of Circle \n Press 2 to calculate area of Rectangle \n Press 3 to calculate area of Square \n Press 4 to Exit"); printf("\n Enter your choice:"); scanf("%d",&choice); switch(choice) { case 1: printf("\n Enter radius:"); scanf("%d",&radius); area=3.14*radius*radius; printf("\n Area of circle: %f",area); break; case 2: printf("\n Enter length:"); scanf("%d",&length); printf("\n Enter breadth:"); scanf("%d",&breadth); printf("\n Area of rectangle: %d",length*breadth); break; case 3: printf("\n Enter Side:"); scanf("%d",&side); printf("\n Area of square: %d",side*side); break; case 4: return 0; default: printf("\n wrong choice"); } return 0; }
Output:
Press 1 to calculate the area of Circle
Press 2 to calculate the area of Rectangle
Press 3 to calculate the area of Square
Press 4 to Exit
Enter your choice:1
Enter radius:5
Area of circle: 78.500000
Menu-driven program in c
Write a c program which performs the following operations:
#include<stdio.h> int main() { char fav; int num = 0, choice = 1, fact = 1, i; printf("\n Enter a Number:"); scanf("%d", &num); do { printf("\n Press 1 to find factorial of a number \n Press 2 to check whether the number is Prime or Not \n Press 3 to find check number is even or odd \n Press 4 to Exit"); printf("\n Enter your choice:"); scanf("%d", &choice); switch (choice) { case 1: for (i = num; i > 1; i--) fact *= i; printf("\n Factorial of %d", num); printf(" is %d", fact); break; case 2: for (i = 2; i < num; i++) { if (num % i == 0) { printf(" \n %d is not a prime number", num); break; } } if (num == i) printf("%d is a prime number", num); break; case 3: if (num % 2 == 0) printf("%d is an even number", num); else printf("\n %d is an odd number", num); break; case 4: return 0; default: printf("\n wrong choice"); } printf("\n Do you want to continue?(y/n)\n"); scanf("\n %c", &fav); } while (fav == 'y'); return 0; }
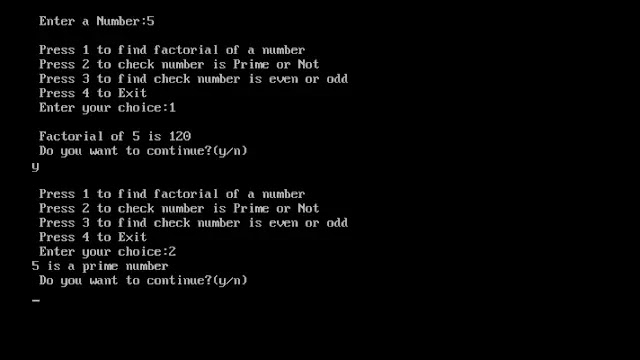

Algorithm for Menu-driven program in c
- Initialize three int variables and a one-character variable.
- Now take the number from the user and store it in an int variable.
- Print all the choices on the screen and take the user's choice.
- We will pass our choice variable to the switch case, and the choice is an int variable containing the user's preference. (you can write any valid identifier)
- Make statements of case 1, case 2, case 3, etc., using for loop and if-else statements, and at the end of every case statement, write a break so that it doesn't go for the next statement.
- Write a default statement in case the user enters the wrong choice.
- Now display a message on the screen that do you want to continue?
- If the user wants to continue, then they should press y.
- Take the input from the user in the character variable, and don't forget to press the space between "and % in the scanf statement.
- In the end, you must write the do-while loop's testing statement.
Menu-driven program for matrix operations in c using switch case
In this program, we will perform all three functions, i.e., addition, subtraction, and multiplication of matrices, using a 2-D array.#include <stdio.h> int main() { int C[10][10], A[10][10], B[10][10], i, j, ch, rows, cols,l; printf("Menu-driven program for matrix operations in c"); printf("\n Enter Rows:"); scanf("%d", &rows); printf("\n Enter Columns:"); scanf("%d", &cols); printf("\n Matrix A\n"); printf("\n Enter the numbers you want to insert in the matrix A:"); for (i = 0; i < rows; i++) { for (j = 0; j < cols; j++) { printf("\t"); scanf("%d", &A[i][j]); } } printf("\n Matrix A:\n"); for (i = 0; i < rows; i++) { for (j = 0; j < cols; j++) { printf("%d", A[i][j]); printf("\t"); } printf("\n"); } printf("\n Enter the numbers you want to insert in the matrix B:"); for (i = 0; i < rows; i++) { for (j = 0; j < cols; j++) { printf("\t"); scanf("%d", &B[i][j]); } } printf("\n Matrix B:\n"); for (i = 0; i < rows; i++) { for (j = 0; j < cols; j++) { printf("%d", B[i][j]); printf("\t"); } printf("\n"); } printf("\n 1. Addition of Matrix A & B "); printf("\n 2. Subtraction Of Matrix A and matrix B n"); printf("\n 3. Multiplication of Matrix A & B"); printf("\n Enter your choice (1 ,2,3):"); scanf("\n %d", &ch); switch (ch) { case 1: printf("\n A+B=\n"); for (i = 0; i < rows; i++) { for (j = 0; j < cols; j++) { C[i][j] = A[i][j] + B[i][j]; printf("%d", C[i][j]); printf("\t"); } printf("\n"); } break; case 2: printf("\n A-B=\n"); for (i = 0; i < rows; i++) { for (j = 0; j < cols; j++) { C[i][j] = A[i][j] - B[i][j]; printf("%d", C[i][j]); printf("\t"); } printf("\n"); } break; case 3: printf("\n A*B=\n"); for (i = 0; i < rows; i++) { for (j = 0; j < rows; j++) { C[i][j] = 0; for (l = 0; l < cols; l++) { C[i][j] = C[i][j] + A[i][l] * B[l][j]; } printf("%d", C[i][j]); printf("\t"); } printf("\n"); } break; default: printf("\n Wrong Choice"); } return 0; }
Output:
Matrix A:
1 2
3 4
Matrix B:
1 2
3 4
1. Addition of Matrix A & B
2. Subtraction Of Matrix A and matrix B
3. Multiplication of Matrix A & B
Enter your choice (1, 2, 3):1
A+B=
2 4
6 8
About this Post:
In this post, we learn to make a menu-driven program to print areas of circles, squares, and rectangles. We also perform matrix operations using a switch case and do-while loop.
The second program answers one of the most essential questions in the book "Let Us C" by Yashwant Singh Kandari.
If you like this, please comment below to support us, and you can freely ask your doubts in the comment section.
This program will clear your concepts about switch case programs, do-while loops, for loops, if-else statements, and exit functions.
The solution of scanf (“%c”,&ch) not working
Important note about taking the value of a character from a user in c using scanf
scanf(“%c”,&ch)
The above statement is wrong, but the compiler ignores this error; when you compile this statement in your program, it will not show any errors.
Moreover, this statement will not be executed when you execute your program.
Therefore you have to press space between "and % means you have to use the following statement:
Moreover, this statement will not be executed when you execute your program.
Therefore you have to press space between "and % means you have to use the following statement:
scanf(“ %c”,&ch)