If you are looking for menu driven program for a calculator in python Click Here
Menu Driven Program in Python using while loop
- Area of Circle
- Area of Rectangle
- Area of square
- Exit
while True: print("Menu Driven Program") print("1.Area of Circle") print("2.Area of Rectangle") print("3.Area of Square") print("4.Exit") choice=int(input("Enter your choice:")) if choice==1: radius=int(input("Enter radius of Circle:")) print("Area of Circle",3.14*radius*radius) elif choice==2: length=int(input("Enter length of Rectangle:")) breadth=int(input("Enter breadth of Rectangle:")) print("Area of Rectangle:",length*breadth) elif choice==3: side=int(input("Enter side of Square:")) print("Area:",side*side) elif choice==4: break else: print("Wrong Choice") repeat=input("Do you want to continue? (y/n)") if repeat=='n'or repeat=='N': break
Menu Driven Program
1. Area of Circle
2. Area of Rectangle
3. Area of Square
4. Exit
Enter your choice:3
Enter the side of Square:5
Area: 25
- Print the menu on the screen.
- Input the choice from the user.
- According to their choice, display the area using the if-else statement.
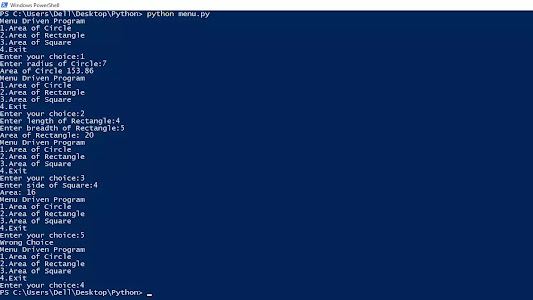
Menu Driven Program for Class 12th Students [CBSE BOARD]
We will make this program using an infinite while loop which will terminate as soon as users choose the option to exit. We will store his choice in a variable, which tells us about his choice so that we can make our if-else statements.Then we ensure that we cover all the conditions that our menu provides. However, there will be another case when the user provides input which is not available in our menu.
For example, you go to Domino and order momos which is unavailable on their menu. So, they will tell you "Wrong choice" and order something else shown on their menu. Hence we will add an else statement that will print the wrong choice.
Video Solution
Menu Driven Program in Python using functions
In this program, we will create a python program for class 12 in which we will calculate the area of different shapes like circles, squares, and rectangles using functions.
def circle(radius): print("Area of Circle",3.14*radius*radius) def rectangle(length,breadth): print("Area of Rectangle:",length*breadth) def square(side): print("Area:",side*side) while True: print("Menu Driven Program") print("1.Area of Circle") print("2.Area of Rectangle") print("3.Area of Square") print("4.Exit") choice=int(input("Enter your choice:")) if choice==1: radius=int(input("Enter radius of Circle:")) circle(radius) elif choice==2: length=int(input("Enter length of Rectangle:")) breadth=int(input("Enter breadth of Rectangle:")) rectangle(length,breadth) elif choice==3: side=int(input("Enter side of Square:")) square(side) elif choice==4: break else: print("Wrong Choice")
Enter your choice:1
Enter radius of Circle:5
Area of Circle 78.5
This Menu-driven program in python using functions is essential for class 12th students. One question about this program can come from the CBSE board, so prepare well for it. If you have any doubt, let me know in the comment section.
We can also call this program a python menu loop because we will display the menu in a loop until the user wants to exit.
Questions Related to this Program
Explain a menu-driven program with an example?
Menu-driven program is a program which will perform operations according to the user's choice from the menu. Here are examples of menu-driven programs.
- To calculate the area/perimeter of different shapes.
- To perform simple arithmetic operations (create a simple calculator) like adding two numbers in python and subtraction.
How do you exit a menu-driven program?
We have to use the break statement to exit from the loop. When users want to not continue, They will choose the option to exit, and in that "if condition," we will write a break statement.
Related post:
Python Program to Check Armstrong Number
Simple Python Program to Print Hello world
Python program to check even or odd
About this program: This is a menu-driven program in python using a while loop and functions. It is an essential menu-driven program for board practicals. You can comment below if you have any queries related to this post.
Please subscribe to our newsletter to connect with us. If you like this post, then please share this post with your friends.